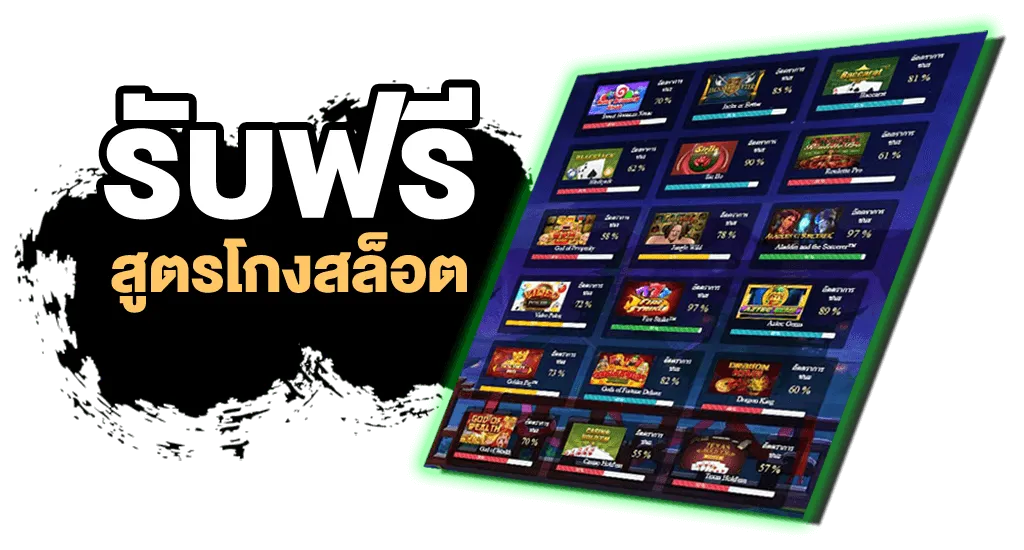
สูตรสล็อต pg ฟรี ใช้ได้ จริง สล็อตที่แม่นยำที่สุดเพื่อช่วยให้คุณเล่นได้ พบกับเกมสล็อต เกมโบนัส แจ็คพอต รับทุกเกมจากทุกค่าย หากต้องการเล่นเกมสล็อต ให้เข้าสู่ระบบผ่านการเข้าสู่ระบบที่แนะนำ เว็บสล็อตโดยตรงไม่ผ่านตัวแทนใช้สูตรการสแกนสล็อตจากผู้ให้บริการและเรา สูตรจะบอกคุณถึงอัตราการชนะ 95% หรือมากกว่า คุณสามารถสร้างรายได้จากการเล่นทุกวัน และเคล็ดลับก็คือแผนภูมิสูตรที่แตกง่ายของเรา
ใครที่อยากใช้สูตรสล็อตฟรีของแต่ละค่ายก็สามารถเริ่มใช้งานได้เลย สูตรpgสล็อต ที่เราคำนวณด้วยระบบ AI 2024 พร้อมใช้งานทันที เริ่มเล่นเกมสล็อตที่คุณชื่นชอบ พร้อมที่จะรับลิงค์แอพเว็บไซต์สล็อตที่แตกได้อย่างง่ายดายรับรายได้จากการเล่นกับเรา ทีมสูตรสล็อตที่แตกได้ นักพัฒนาซอฟต์แวร์สูตรสล็อตฟรี ทดสอบแล้วว่าใช้งานได้จริง มีความแม่นยำถึง 98%
เกมสล็อตออนไลน์ PG SLOT เป็นเกมสล็อตในโลกออนไลน์ ได้รับความนิยมมากที่สุดในช่วงปี 2021 – 2024 สูตรสล็อต pg ฟรี ใช้ได้ จริง 2024 ล่าสุด และวิธีการเล่นที่เป็นนวัตกรรมและทำจากเกมอาร์เคดยอดนิยม เพื่อให้ทันสมัยยิ่งขึ้น
และเนื่องจากเป็นเหตุผลยอดนิยมในการดึงดูดผู้เล่นจำนวนมากเข้ามาและคว้าถ้วยรางวัล และมาทำกำไรซึ่งให้คำแนะนำที่ดีในการเล่นบริการสูตรสล็อต pg ที่จะช่วยผู้เล่นทุกคน รับสิทธิประโยชน์ต่อเนื่อง สร้างง่าย ได้เงินจริง เราขอท้าให้คุณพิสูจน์ ลองเล่นสล็อตฟรีกับเราได้แล้ววันนี้ เว็บไซต์สล็อตคุณภาพ ได้เงินเร็ว ไม่ผิดหวังแน่นอน
ขณะนี้เราเชื่อว่าคงไม่มีผู้เล่นคนใดเลย ไม่คุ้นเคยกับโปรแกรมสูตรสล็อต เพราะสิ่งที่เรากำลังพูดถึง เป็นตัวช่วยที่สำคัญ ของการเล่นสล็อตออนไลน์ ทำให้ได้เงินรางวัลเกมง่ายขึ้นโดยไม่ต้องเสี่ยงกับความยุ่งยากในการเล่นสล็อต เวลาไหนดี และสำหรับผู้เล่นคนไหน ที่ต้องการโปรแกรมสูตรสล็อตกับเว็บไซต์ของเราก็ทำได้ง่ายๆ
สมัครสมาชิก
ฝาก ถอน
ความปลอดภัย
สูตร pg slot ฟรีใช้ได้จริงและกำลังเป็นที่นิยมในเวลานี้ มีเกมให้เลือกเล่นมากมาย หลายค่ายรวมกันที่เฉพาะเกมที่ให้บริการได้รับความนิยมอย่างมากและทำเงินได้มากที่สุดฉันต้องให้ที่นี่ มีเกมสล็อตที่เล่นง่าย สล็อตแตกบ่อยที่สุด ได้รับการพัฒนาให้ทันสมัยและสะดวกสบายให้ผู้เล่นเล่นได้สะดวกและง่ายดายยิ่งขึ้น สำหรับมือใหม่เล่นแล้วได้กำไรทันที
นอกจากนี้ ยังมีสูตรสล็อต pg ฟรี ใช้ได้ จริง แจกฟรี รับรองว่าแม่นที่สุด ฝาก-ถอน เงินง่ายด้วยระบบอัตโนมัติ ไม่มีขั้นต่ำซึ่งเป็นคุณสมบัติที่น่าสนใจ หากคุณมาใช้บริการกับเว็บไซต์เล่นสล็อตที่ครอบคลุมที่สุด มีหลายวิธีในการเล่นเลือกเล่นผ่านเว็บเบราว์เซอร์ โดยตรงไม่จำเป็นต้องติดตั้งหรือดาวน์โหลดแอปในเครื่องเล่นให้เสียเวลาอีกต่อไป
นอกจากนี้ยังมีบริการโปรโมชั่นโบนัสอีกมากมายให้เลือกฟรีทุกวัน แค่เป็นสมาชิกก็คุ้มค่ากับความพยายามแล้ว ไม่ต้องฝากและรับสูตรสล็อต pg ฟรีที่สามารถใช้งานได้จริง เพียงลงทะเบียน ติดง่ายไม่ยุ่งยาก เข้าสู่ระบบเพื่อเล่น ทุกคนจะได้สัมผัสประสบการณ์การเล่นที่คุ้มค่าที่สุดในแต่ละบริการ จะต้องสมัคร สูตรpgสล็อต ที่นี่เท่านั้น
คนส่วนใหญ่อาจจะคุ้นเคยกับสูตรสล็อต pg ฟรีที่สามารถใช้ได้จริงหรือโปรแกรมสแกนคีย์ โดยสามารถรับได้ฟรี ไม่ต้องเสียเงินแม้แต่บาทเดียวในการซื้อ เพียงสมัครสมาชิกก็สามารถร่วมสนุกไปกับเกมคีย์ออนไลน์ด้วยสูตรคีย์ที่จะช่วยให้คุณใช้มันเอาชนะเกมและมีโอกาสลุ้นรับรางวัลโบนัสก้อนใหญ่
สูตรคีย์ pg ฟรีสามารถใช้ได้จริงซึ่งสามารถทำได้ ใช้พัฒนาจากระบบที่แม่นยำยิ่งขึ้น AI ซึ่งจะช่วยคำนวณโอกาสในการชนะมากกว่าแพ้อย่างแน่นอน ดังนั้นใครที่กำลังมองหาสูตรช่วยเล่นและสร้างรายได้บนเว็บไซต์ สล็อต PG ที่แตกง่าย ควรลองใช้สูตรคีย์ PG ฟรีที่ใช้งานได้จริงซึ่งมีความสำคัญสูงสุด สามารถใช้เล่นและลงทุนสูงอย่างต่อเนื่องได้เช่นกัน สูตรสล็อต pg ฟรี ใช้ได้ จริง